0. Redux의 핵심 개념
Store
- 상태가 관리되는 오직 하나의 공간
- 컴포넌트와는 별개로, 스토어 안에 앱에서 필요한 상태를 저장
- 컴포넌트에서 상태 정보가 필요할 때 스토어에 접근
- getState(): 현재 state를 가져오는 메소드
- subscribe(): 변화를 감지하는 메소드
Action
- 앱에서 스토어에 운반할 데이터
- 객체 형식
{
type: 'ACTION_CHANGE_USER', // 필수
payload: { // 옵션
name: 'myname',
age: 25
}
}
Reducer
- 데이터를 변경하는 함수
- Action -> Reducer -> Store
- Action을 Reducer에 전달하기 위해서는 dispatch() 메소드 사용
- dispatch(): Reducer에게 보내는 메시지
- Reducer가 주문을 보고 Store의 상태를 업데이트
- Reducer가 return하는 값 = Application의 state
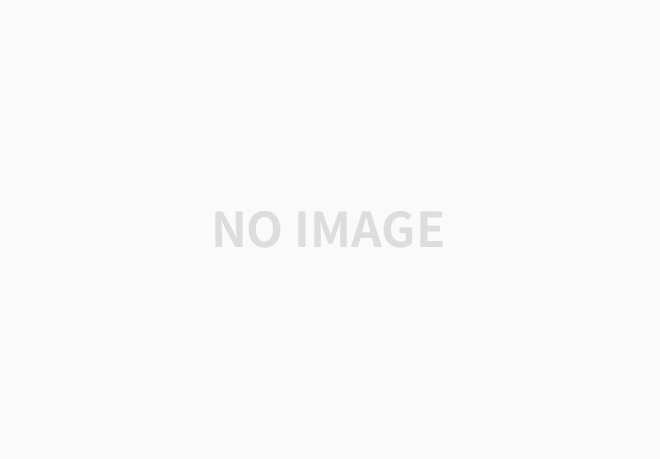
1. Vanilla Counter
const add = document.getElementById("add");
const minus = document.getElementById("minus");
const number = document.querySelector("span");
let count = 0;
number.innerText = count;
const updateText = () => {
number.innerText = count;
}
const handleAdd = () => {
count = count + 1;
updateText();
};
const handleMinus = () => {
count = count - 1;
updateText();
};
add.addEventListener("click", handleAdd);
minus.addEventListener("click", handleMinus);
- 화면에서 count 값을 수정하기 위해 handle~()이 클릭될 때마다 updateText() 함수를 호출하고 있다.
- count++과 count--는 한 번 일어나기 때문에!
- => html에게 수정 사항을 함수로 알려준다.
2. Store and Reducer
- npm/yarn install redux
- redux를 설치한다!
import {createStore} from redux;
const reducer = () => {};
const store = createStore(reducer);
- store는 data를 넣는 곳으로 data를 넣을 수 있는 장소를 만든다.
- data는 state를 의미하며, state는 application에서 바뀌는 data이다.
- Redux에는 createStore() 함수로 store를 만들 수 있다.
- store에는 reducer() 함수를 전달해야 하고, reducer()는 data를 수정한다.
- reducer가 return하는 것은 application의 data가 된다.
const countModifier = (state = 0) => {
... modify ...
return state;
};
const countStore = createStore(countModifier);
- store를 만들면 받은 reducer를 initial state로 불러온다.
- state는 undifined이나, 디폴트 값을 설정할 수 있다.
3. Actions
const countModifier = (state = 0, action) => {
if (action.type === "ADD") {
return count + 1;
} else if (action.type === "MINUS") {
return count - 1;
} else {
return count;
}
return state;
};
countStore.dispatch({type: "ADD"});
countStore.dispatch({type: "MINUS"});
- action을 사용해 수정한다.
- action은 redux에서 함수를 부를 때 쓰는 두 번째 parameter이다.
- createStore.dispatch() 함수로 action을 보내는데, action은 type이 있어야한다.
- 한마디로, dispatch와 함께 reducer로 메시지를 보내는 것이다.
4. Subscriptions
const onChange = () => {
number.innerText = countStore.getState();
}
countStore.subscribe(onChange);
- createStore.subscribe() 함수는 store 안의 변화를 보여준다.
- store에 변화가 있을 때마다 감지해서 불려진다.
5. Recap and Refactor
const ADD = "ADD";
const MINUS = "MINUS";
const countModifier = (state = 0, action) => {
switch (action.type) {
case ADD:
return count + 1;
case MINUS:
return count - 1;
default:
return count;
}
};
- string이 아닌 const로 변수를 만들어 사용할 시 오류를 찾기에 용이하다.
- if-else 문보다 switch 문이 간편하다.
'Group Study (2022-2023) > React.js' 카테고리의 다른 글
[React] 4주차 스터디 - Handling Events & react-router-dom (0) | 2022.11.06 |
---|---|
[React] 3주차 스터디 - (심화) Pure Redux(2): To-do List (0) | 2022.10.30 |
[React] 3주차 스터디 - (입문) React의 Hooks (0) | 2022.10.28 |
[React] 2주차 스터디 - (입문) React의 핵심 개념 익히기 (컴포넌트, Props, State, Lifecycle) (0) | 2022.10.09 |
[React] 1주차 스터디 - React 프로젝트 생성하기 & JSX / Redux 시작하기 (0) | 2022.10.02 |