Android
안드로이드는 구글에서 만든 스마트폰용 운영체제이다. 리눅스(Linux)기반이며 자바를 사용한다.
1. TextView
TextView는 화면에서 Text를 표시할 때 사용하는 뷰위젯이며, Button 과 EditText의 부모 클래스이다.
- Button, EditText 의 부모 클래스 -> TextView 의 부모 클래스 -> View 클래스
<TextView
android:layout_marginTop="70dp"
android:layout_gravity="center_horizontal"
android:layout_width="wrap_content"
android:textAlignment="center"
android:layout_height="wrap_content"
android:text="Get the life you want!\n
Save more money."
android:textColor="#ffffff"
android:textSize="20dp"
android:textStyle="bold"
/>
이번 실습 과제였던 LayoutPractice의 일부이다.
TextView는 안드로이드 프로젝트를 처음 만들었을 때 생기는 xml 레이아웃 리소스에도 표시된다. TextView에는 text와 관련된 다양한 속성들이 존재한다. 대표적으로
android:text | TextView에 text를 설정한다. |
android:gravity | TextView의 Text를 수직, 수평, 혹은 둘다의 형태로 정렬한다. |
android:height | TextView의 높이를 설정한다. |
android:width | TextView의 넓이를 설정한다. |
android:hint | Text가 없을 때 hint를 표시한다. ex) 아이디를 입력하세요 |
android:textColor | text의 색을 설정한다. |
android:textSize | text의 크기를 설정한다. |
android:textStyle | text의 스타일을 설정한다. ex) bold, italic, bolditalic |
2. EditText & Button
1) EditText
- TextView를 상속받는 클래스이다. TextView는 단순히 Text를 보여주는 역할이라면, EditTExt는 Text를 입력 및 수정까지 가능한 뷰위젯이다.
- enabled 속성을 사용하면 EditText의 텍스트를 입력 및 수정이 불가능하게 설정할 수 있다. default는 true이다.
2) Button
- TextView를 상속받는 클래스이다. 사용자가 탭하거나 클릭하는 액션을 수행하도록 하는 사용자 인터페이스 요소이다.
- styling하지 않은 button color의 default color는 보라색이다.
<android.widget.Button
android:layout_width="match_parent"
android:layout_height="60dp"
android:text="Create free account"
android:textAllCaps="false"
android:textColor="#fff"
android:textSize="20dp"
android:background="@drawable/rounded_corner"
/>
이번 실습 과제였던 LayoutPractice의 일부이다. android:background 에 drawable폴더의 rounded_corner.xml을 참조하여 색과 border을 설정했다.
3. ImageView & Toast
1) ImageView
- View 클래스를 상속받는다.
- 이미지 리소스를 보여준다. (ex. Bitmap 이나 Drawable 리소스) 또한, 이미지를 꾸미거나 image scaling을 다룰 수 있다.
2) Toast
- 작은 팝업으로 작업에 관한 간단한 피드백을 제공한다. 메시지에 필요한 공간만 차지하며 진행 중인 활동은 그대로 효시되고 상호작용도 유지된다. 일정 시간이 지나면 자동으로 사라진다.
- Android(APi 수준31) 이상 타켓팅이라면 텍스트 두줄 제한이다. 줄 길이는 화면 크기에 따라 다르므로 텍스트를 최대한 짧게 만드는 것이 좋다.
val text = "Hello toast!"
val duration = Toast.LENGTH_SHORT
val toast = Toast.makeText(this, text, duration) // in Activity
toast.show()
다음 매개변수를 갖는 makeText() 메서드를 사용한다.
- 활동 Context
- 사용자에게 표시되어야 하는 텍스트
- 토스트 메시지가 화면에 남아 있어야 하는 시간
4. LinearLayout, RelativeLayout, ConstraintLayout
1) LinearLayout
- LinearLayout은 세로 또는 가로의 단일 방향으로 모든 하위 요소를 정렬하는 뷰 그룹이다.
- android:orientation 속성으로 레이아웃 방향을 지정할 수 있다.
- android:layout_weight: 개별 하위 요소에 가중치를 할당할 수 있다. (HTML의 flexbox와 비슷) 해당 뷰에 '중요도' 값을 할당한다. 큰 가중치 할당 -> 남은 공간 가중치 비율에 따라 할당
LinearLayout에 android:weightSum을 3으로하고 각각 하위 view 클래스에 layout_weight을 1씩 줬다.
2) RelativeLayout
- 상대 위치에 하위 뷰를 표시하는 뷰 그룹이다. 각 뷰의 위치는 동위 요소(다른 뷰의 왼, 또는 아래)에 상대적이거나 상위 RelativeLayout 영역에 상대적인 위치로 지정할 수 있다.
- 뷰끼리 겹칠 수 있다.
android:layou_alignParentTop | true면 이 뷰의 상단 가장자리를 상위 뷰의 상단 가장자리와 일치 시킨다. |
android:layout_centerVertical | true면 이 하위 요소를 상위 요소 내에 세로로 중앙에 배치한다. |
android:layout_below | 이 뷰의 상단 가장자리를 리소스 ID로 지정한 뷰 아래에 배치한다. |
android:layout_toRightOf | 이 뷰의 왼쪽 가장자리를 리소스 ID로 지정된 뷰의 오른쪽 에 배치한다. |
다음과 같이 뷰끼리 겹칠 수도 있다. 주황색 박스는 width를 match_parent (자신의 부모에 폭이나 넓이를 맞춤)로 하고 작은 박스들은 width, height 모두 50dp이다.
3) ConstraintLayout
- ConstraintLayout 플랫 뷰 계층 구조로 중첩이 없다. 크고 복잡한 레이아웃을 만들 수 있다.
- RelativeLayout보다 유연하다.
- 화면전환이 이루어졌을 때 비율을 유지한다.
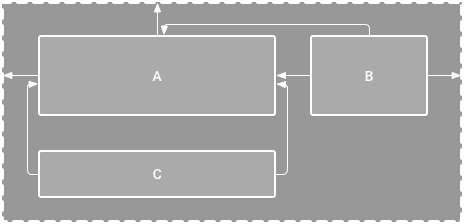
그림 1. 보기 C가 A 아래에 표시되지만 세로 제약조건이 없는 편집기
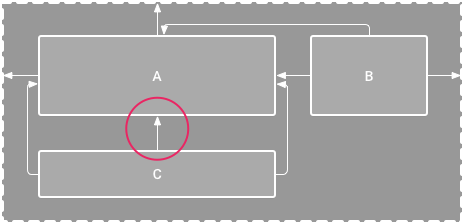
그림 2. 보기 A 아래에 세로로 제한된 보기 C
그림 1의 편집기에서 보기 C에 세로 제약조건이 없다. 기깅 이 레이아웃을 그리면 보기 C가 보기 A의 왼쪽과 오른쪽 가장자리에 맞게 가로로 정렬되지만, 세로 제약조건이 없으므로 화면의 맨 위에 표시된다.
<참고>
https://www.geeksforgeeks.org/textview-widget-in-android-using-java-with-examples/https://lktprogrammer.tistory.com/141
https://developer.android.com/guide/topics/ui/controls/button?hl=kohttps://developer.android.com/reference/android/widget/ImageView
https://developer.android.com/guide/topics/ui/notifiers/toasts?hl=ko#kotlin
'Group Study (2023-2024) > Android 입문' 카테고리의 다른 글
[Android 입문] 5주차 스터디 - RoomDB, SharedPreferences, Datastore (0) | 2023.12.25 |
---|---|
[Android 입문] 4주차 스터디 -Viewpager2, TabLayout, Navigation View (0) | 2023.11.29 |
[Android 입문] 3주차 스터디 -ListView, RecyclerView, ListView와 RecyclerView의 차이점 (1) | 2023.11.20 |
[Android 입문] 2주차 스터디 -Activity와 Fragment, ViewBinding (0) | 2023.11.13 |